You can read about the newer version of the Bluetooth network monitor here, but I suggest you continue reading this article.
I often encounter slow download speeds or online gaming lag, and every time I have to log in to my router’s web page or via SSH to check if it’s because of others in my household downloading/uploading, unstable network connections, or simply a server issue. It’s really frustrating. So, I decided to create a device that displays network statistics in real-time, making it more convenient to monitor network traffic.
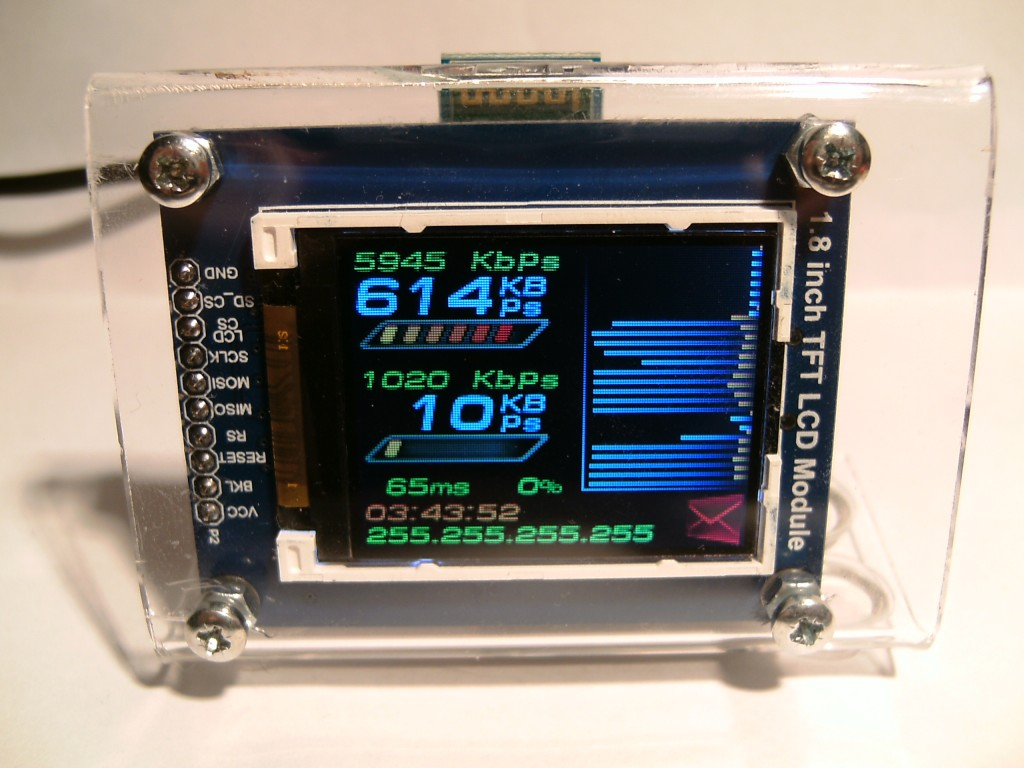
The monitor consists of an ATmega328P @ 20MHz, a 160×128 1.8-inch color LCD, and a Bluetooth module, powered by 5V USB. It has a button that long-presses to adjust the LCD brightness and short-presses to switch display modes. Since it’s powered by USB, I added support for V-USB.
Enclosure Design:
- Uses an A8 paper display holder
- Removes the back clip part
- Adds rubber feet
Monitor Modes:
- Display Mode 1:
- Downlink sync
- Uplink sync
- Download rate
- Upload rate
- 25-second historical chart
- Flat
- Packet loss
- WAN IP
- Time
- Email notification
- Display Mode 2:
- Download rate
- Upload rate
- 40-second large historical chart
Future Plans:
- Add more display modes, such as using V-USB to display CPU usage from PC power supply, etc.
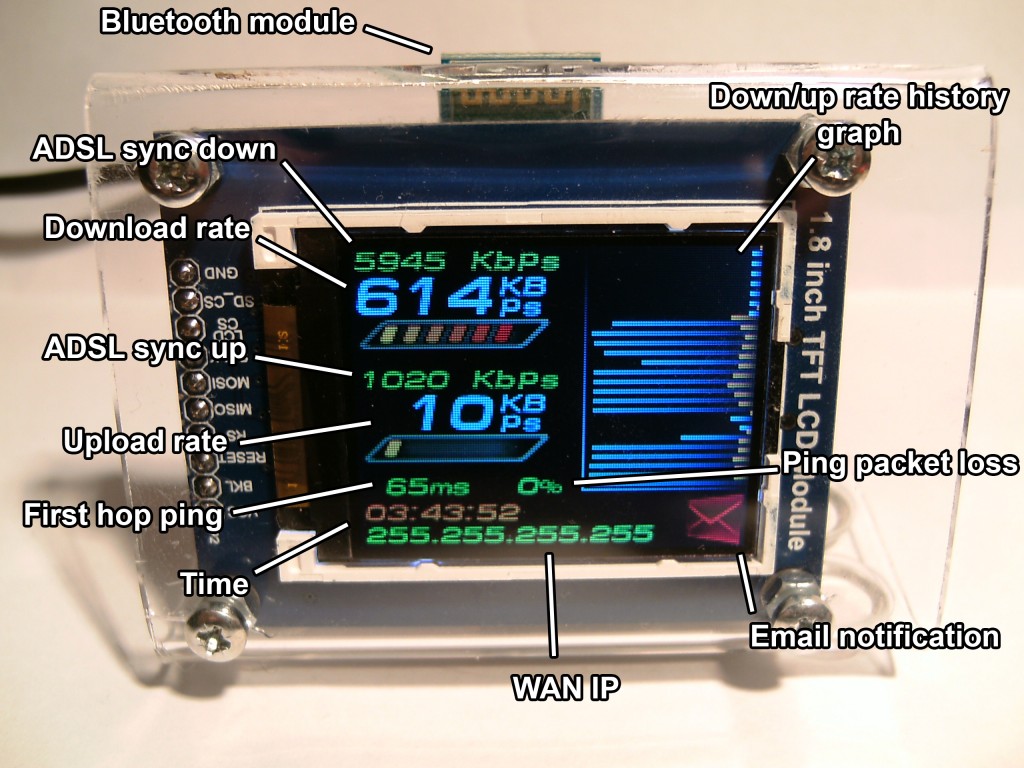
The router is a PC running Debian Linux, which uses a shell script to obtain information such as download and upload speeds, ping, packet loss, WAN IP, and other data from the WAN interface. The script then sends this data to a serial port, which is connected to a serial Bluetooth module, and finally displays the data on an LCD screen.
To adjust the modem’s synchronization speed, you need to run a CGI script on the modem to parse the ADSL status page. However, since you are running OpenWRT on a Netgear DG834Gv3, you can write a small shell script to obtain the ADSL status from the /proc
file, without needing to parse the HTML status page.
Here is a possible solution:
- Write a shell script on the router to obtain information such as download and upload speeds, ping, packet loss, WAN IP, and other data from the WAN interface using tools like
curl
orwget
. - Use a serial port tool like
screen
orminicom
to send the obtained data to the serial port. - Write a small program on the serial Bluetooth module to receive data from the serial port and send it to another Bluetooth module on the network monitor.
- Write a small program on the Bluetooth module on the network monitor to receive data from the serial port and display it on the LCD screen.
- To adjust the modem’s synchronization speed, write a small shell script to obtain the ADSL status from the
/proc
file, parse the obtained information, and adjust the synchronization speed as needed.
Optimization and rewriting ideas include:
- Using a more efficient serial communication protocol, such as RS-232 or TCP/IP, to increase data transfer speed.
- Using a lighter-weight shell scripting language, such as bash or ash, to reduce system resource usage.
- Implementing a caching mechanism to store obtained data, reducing the number of accesses to the serial port.
- Using a more efficient Bluetooth module communication protocol, such as SPP or HID, to increase data transfer speed.
Note that this is just one possible solution, and you may need to adjust and optimize it according to your specific situation.
Protocol
The protocol used is a very simple text based one$|syncdown|syncup|ratedown|rateup|ping|loss|ip|emails|date|time|*
Example:$|6100|1020|340|4|19|0|255.255.255.255|2|Wed 02 Jan 13|20:31:26|*
Special characters:
$ – Start of packet
\* – End of packet
| – Delimiter to separate pieces of data
other
When you have spare time, using C language to write programs instead of shell scripts can reduce CPU usage and make it easier to use more efficient binary protocols. This is a very good approach, especially if you need to optimize performance-critical parts of your system.
By using C language to write programs, you can:
- Reduce CPU usage and avoid shell script interpretation overhead
- Use more efficient binary protocols for communication
- Optimize memory usage and reduce latency
- Utilize low-level system APIs and libraries
This is especially important if you are working on resource-constrained systems or real-time applications, where every millisecond counts.
I’m glad to hear that you’re making progress with using SMD components (0805 size)! Using SMD components can be challenging, especially for beginners, but with practice, you’ll become more confident and proficient.
In future projects, you may consider using SMD components more extensively, especially if you’re working on compact or low-power designs. Just remember to follow proper soldering techniques and use the right tools to ensure reliable connections.
For future projects, you may consider the following optimization and rewriting strategies:
- Use modular design principles to break down complex systems into smaller, more manageable components
- Optimize algorithms and data structures to improve performance and efficiency
- Use Profiling tools to identify performance bottlenecks and optimize accordingly
- Consider using more efficient communication protocols and data formats
- Utilize parallel processing and multi-threading to accelerate computationally intensive tasks
By following these strategies, you can create more efficient, reliable, and scalable systems that meet your performance requirements.
I also made a small utility for converting the red channel of images into a byte array representing the intensity of each pixel, colour is added on-the-fly by the the controller based on the intensity, allowing multiple colours of the same image or font without using up extra flash space.
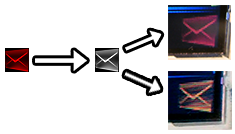
All the rendering takes quite a bit of processing time which is why the the chip is running at 20MHz, compiler settings and which compiler that is used also effects the performance, going from Atmel Studio 6’s default settings to using AVR-GCC 4.7.2, using these flags for the C compiler –-O2 -fno-tree-scev-cprop -ffreestanding -mcall-prologues
and these flags for the linker –-mrelax
reduces program size by around 2KB (now 16,836 bytes), saves 18 byes of RAM (now 301 bytes, that doesn’t count runtime stuff which tops at around 1KB) and gains a few extra FPS.
Using -Os
instead of -O2
would give an even smaller code size, but at the cost of performance.
Sources available at GitHubhttps://www.youtube.com/embed/F0jkh3hcmN8
Video of the net monitor in early development – https://www.youtube.com/watch?v=W9UXh1g5AcA
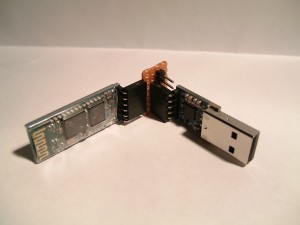
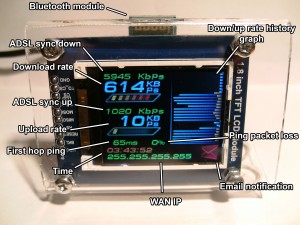
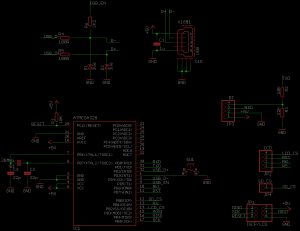
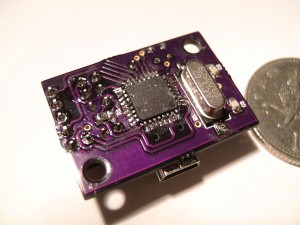
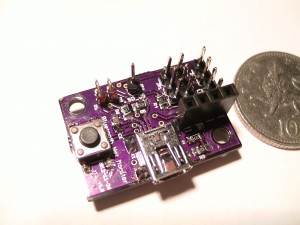
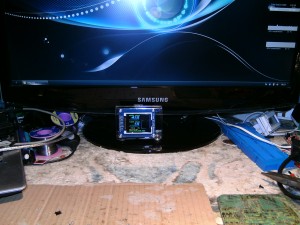
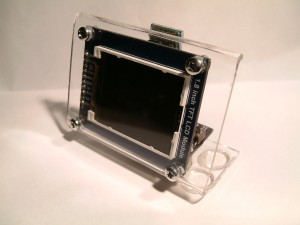
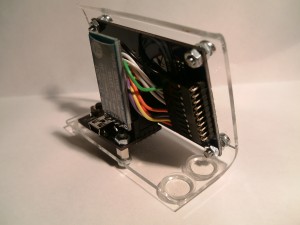
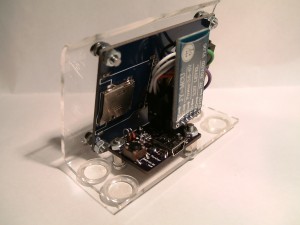
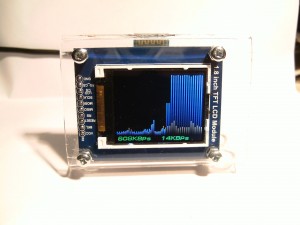
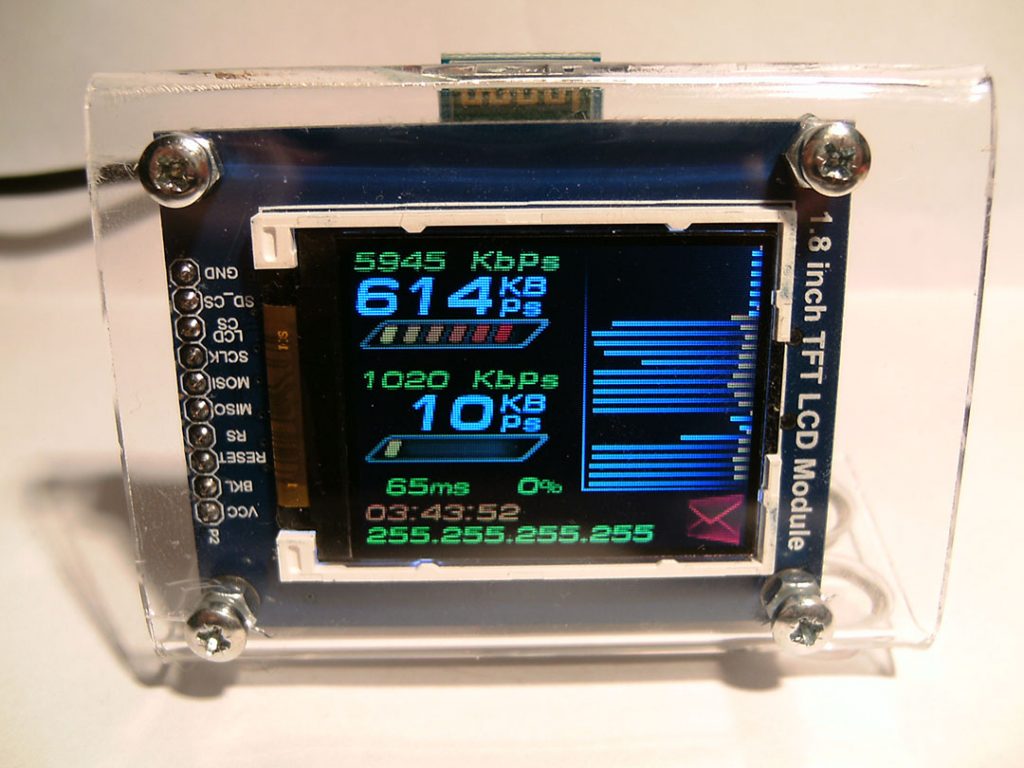